Are you tired of writing clunky, inefficient Python code? 🐍 Do you dream of becoming a coding wizard, capable of crafting elegant and powerful programs with ease? Look no further! We're about to unveil10 Python tricks that will transform your coding skills and elevate you to programming greatness.
In the world of Python, there's always more to learn. Even seasoned developers can benefit from discovering new techniques and shortcuts. Whether you're a beginner looking to level up or an experienced coder seeking to refine your craft, these tricks will add a touch of magic to your code. From mastering list comprehensions to embracing Pythonic idioms, we'll explore a treasure trove of tips that will make your code more efficient, readable, and downright impressive.
Get ready to dive into the enchanting realm of Python wizardry! We'll journey through advanced concepts like generators, decorators, and context managers, unlocking the full potential of this versatile language. By the end of this post, you'll be armed with powerful tools to tackle any coding challenge. So, grab your wand (or keyboard) and let's embark on a magical adventure through these 10 Python tricks that will elevate your coding prowess to new heights! 🧙♂️✨
Mastering List Comprehensions
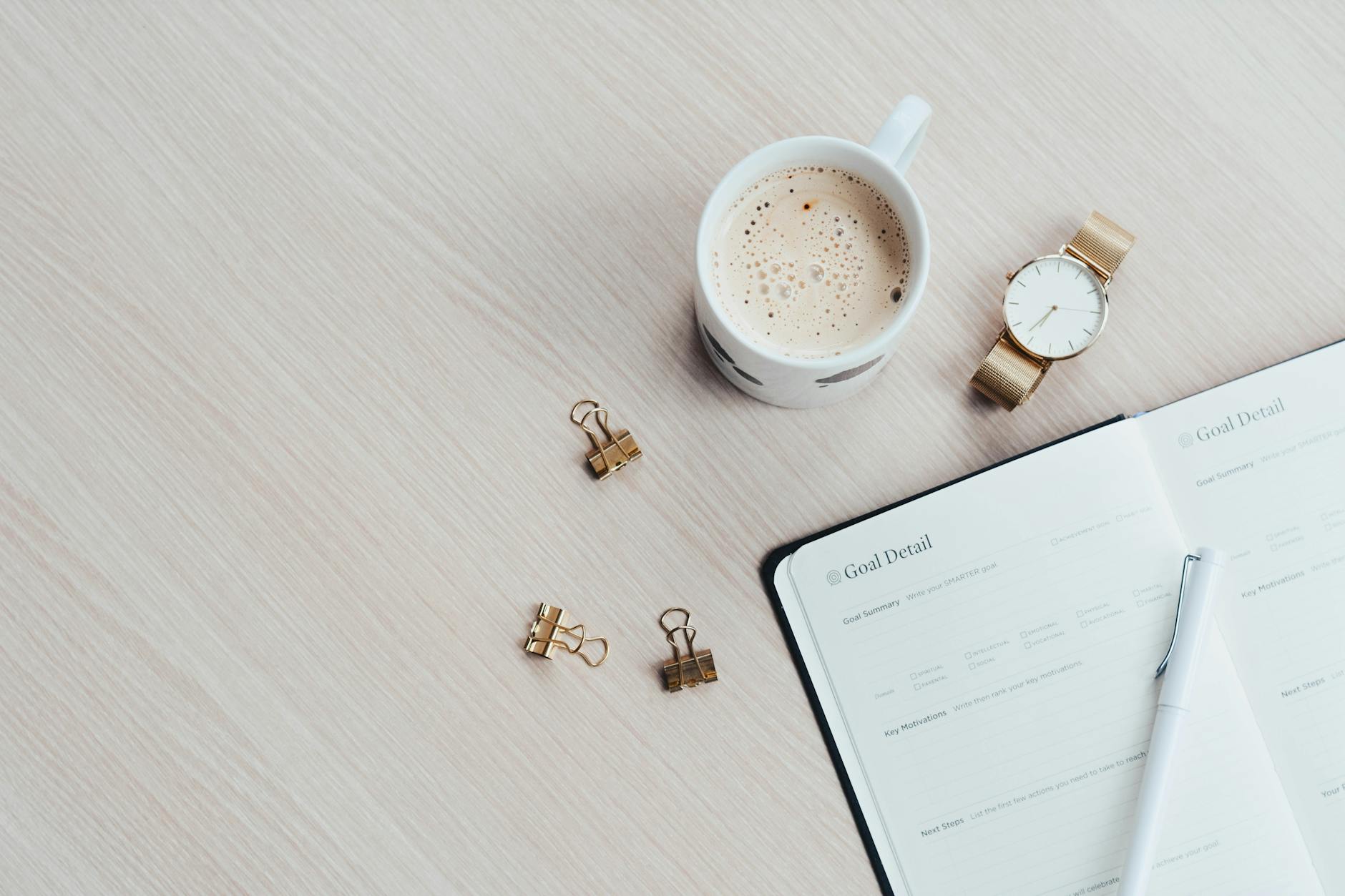
Simplify complex loops
List comprehensions are a powerful Python feature that can significantly simplify your code. They allow you to create lists in a concise and readable manner, often replacing multiple lines of traditional for loops with a single line of code. Here's how you can use list comprehensions to simplify complex loops:
# Traditional loop
squares = []
for i in range(10):
squares.append(i**2)
# List comprehension
squares = [i**2 for i in range(10)]
Create nested list comprehensions
Nested list comprehensions take this concept further, allowing you to create multi-dimensional lists with ease. They can be particularly useful when working with matrices or processing nested data structures:
# Create a 3x3 matrix
matrix = [[i*j for j in range(3)] for i in range(3)]
Here's a comparison of nested list comprehensions vs. traditional loops:
Method | Code Complexity | Readability | Performance |
---|---|---|---|
Nested Loops | Higher | Lower | Slower |
List Comprehension | Lower | Higher | Faster |
Filter data efficiently
List comprehensions excel at filtering data. By incorporating conditional statements, you can create powerful filters that select specific elements from a list:
-
Filter even numbers:
[x for x in range(20) if x % 2 == 0]
-
Filter strings by length:
[word for word in words if len(word) > 5]
List comprehensions not only make your code more concise but also more efficient. They are optimized by Python's interpreter, often resulting in faster execution compared to traditional loops. As you continue to explore Python's features, you'll find that mastering list comprehensions is a crucial step towards becoming a more proficient programmer.
Leveraging Lambda Functions
Write concise one-line functions
Lambda functions in Python are a powerful tool for creating concise, one-line functions. These anonymous functions can be used to simplify your code and make it more readable. Here's how you can leverage lambda functions effectively:
-
Basic syntax:
lambda arguments: expression
-
Use cases:
-
Simple calculations
-
Filtering data
-
Transforming data
-
Example:
# Traditional function
def square(x):
return x ** 2
# Lambda equivalent
square = lambda x: x ** 2
Use lambda with map() and filter()
Lambda functions shine when used in combination withmap()
andfilter()
:
Function | Purpose | Example |
---|---|---|
map() | Apply a function to each item in an iterable | list(map(lambda x: x * 2, [1, 2, 3])) |
filter() | Filter items based on a condition | list(filter(lambda x: x % 2 == 0, [1, 2, 3, 4])) |
Enhance sorting with custom keys
Lambda functions are particularly useful when sorting complex data structures:
# Sorting a list of tuples by the second element
data = [(1, 'b'), (3, 'a'), (2, 'c')]
sorted_data = sorted(data, key=lambda x: x[1])
By mastering lambda functions, you'll be able to write more efficient and elegant Python code. Next, we'll explore the power of generators, which can help you optimize memory usage in your programs.
Harnessing the Power of Generators
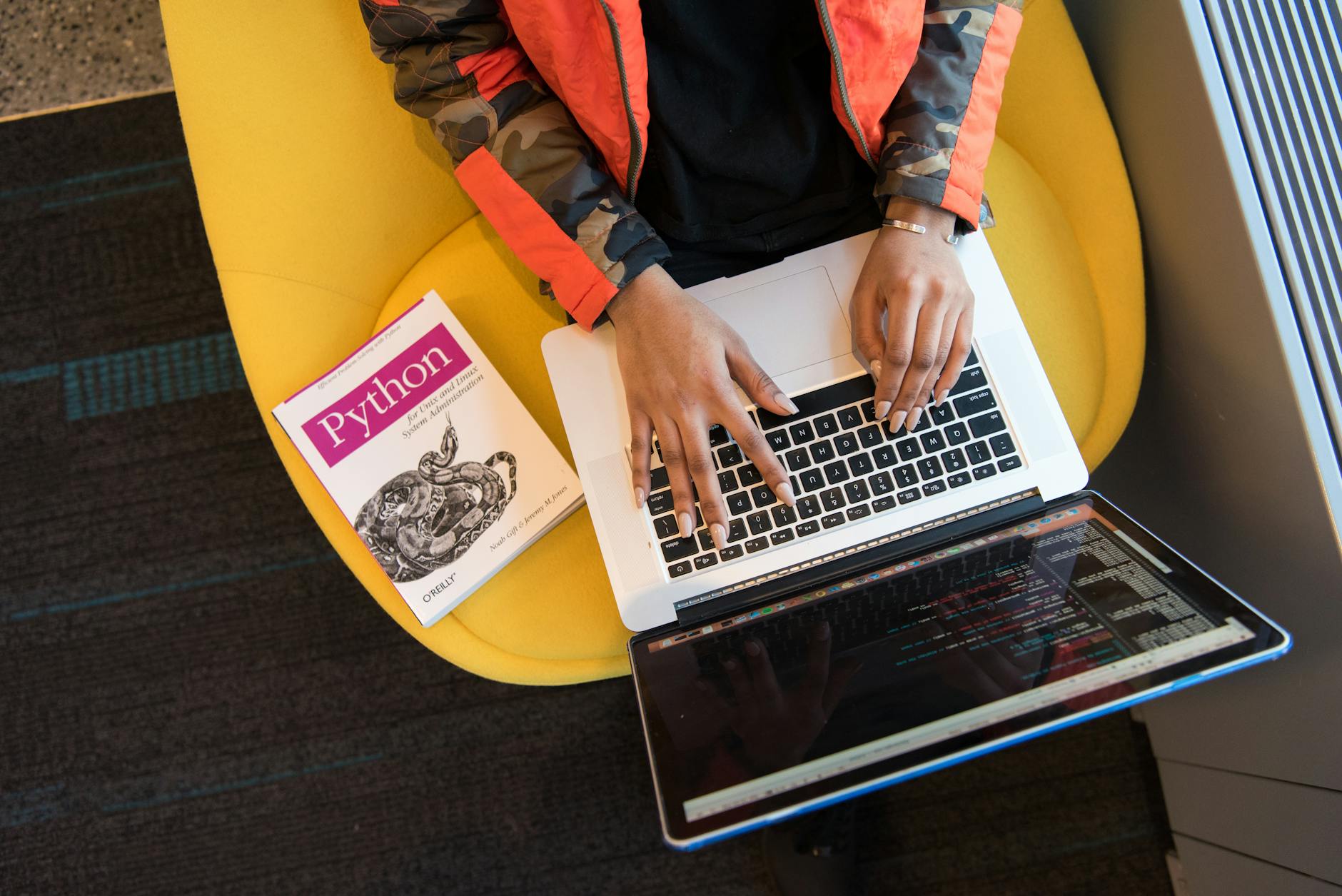
Save memory with lazy evaluation
Generators in Python are a powerful tool for efficient memory usage through lazy evaluation. Unlike lists that store all elements in memory at once, generators produce values on-the-fly, making them ideal for handling large datasets or infinite sequences.
Here's a comparison between a list and a generator:
Feature | List | Generator |
---|---|---|
Memory Usage | Stores all elements | Generates values on-demand |
Speed | Faster for small datasets | More efficient for large datasets |
Iteration | Can be iterated multiple times | Can be iterated only once |
To create a generator, you can use either generator expressions or generator functions:
-
Generator expression:
squares = (x**2 for x in range(1000000))
-
Generator function:
def squares(): for x in range(1000000): yield x**2
Create infinite sequences
Generators excel at creating infinite sequences without consuming infinite memory. This is particularly useful for mathematical sequences or simulations.
Here's an example of an infinite Fibonacci sequence generator:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
Build custom iterators
Generators simplify the process of building custom iterators. Instead of implementing__iter__()
and__next__()
methods, you can use a single generator function.
Example of a custom date range iterator:
from datetime import date, timedelta
def date_range(start, end):
current = start
while current <= end:
yield current
current += timedelta(days=1)
Now that we've explored generators, let's move on to another powerful Python feature: decorators.
Utilizing Decorators for Code Enhancement
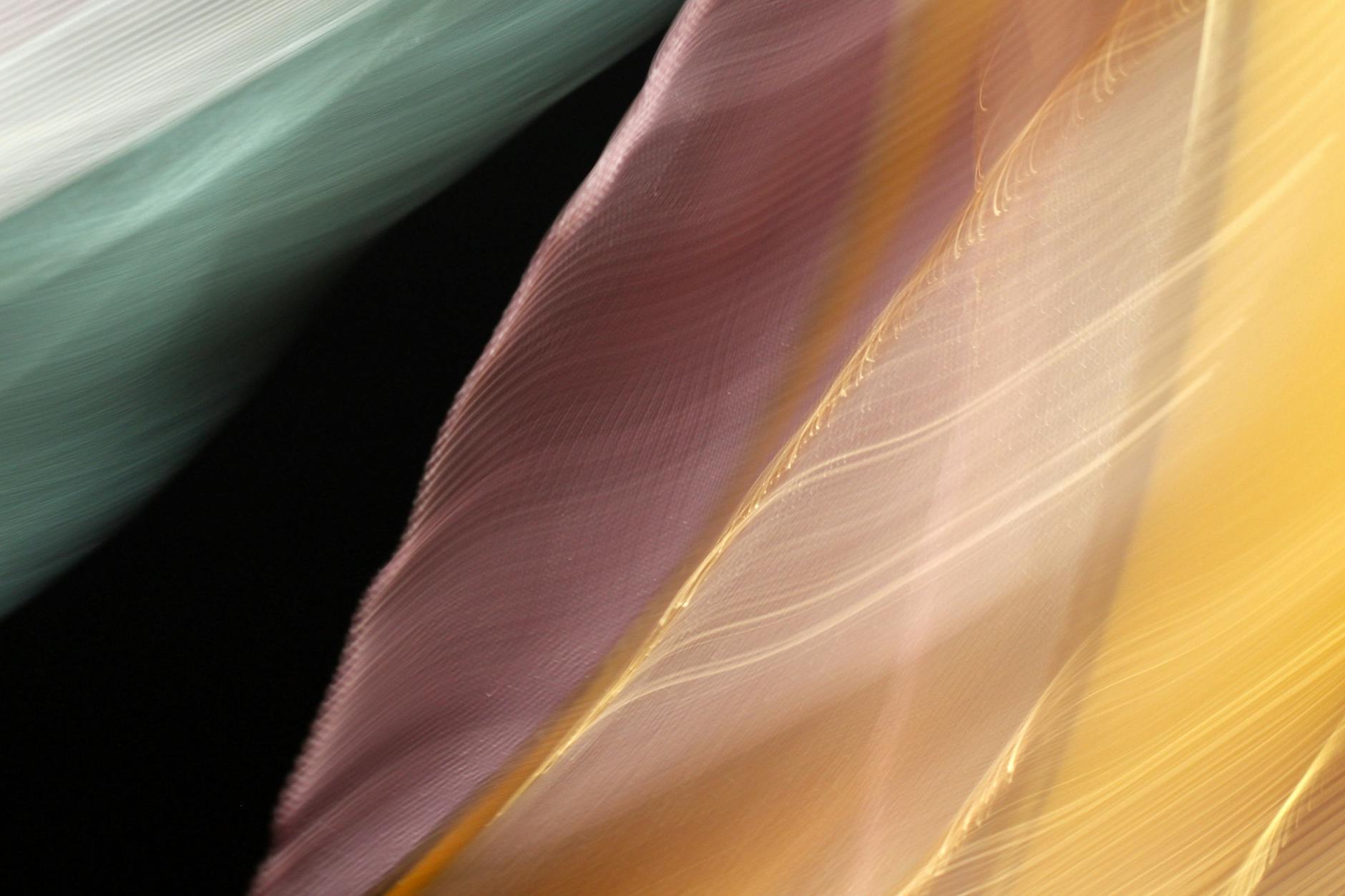
A. Modify function behavior without changing its code
Decorators in Python offer a powerful way to modify function behavior without altering its source code. This capability allows for clean, modular, and reusable code. Let's explore how decorators can enhance your functions:
-
Basic decorator structure:
def my_decorator(func): def wrapper(): # Do something before result = func() # Do something after return result return wrapper @my_decorator def my_function(): pass
-
Use cases for modifying function behavior:
-
Input validation
-
Caching results
-
Authentication and authorization
-
Retry mechanisms
-
B. Implement timing and logging decorators
Timing and logging decorators are excellent examples of how to enhance code functionality without cluttering the main logic. Here's how you can implement them:
import time
import logging
def timer_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute")
return result
return wrapper
def logging_decorator(func):
def wrapper(*args, **kwargs):
logging.info(f"Calling {func.__name__}")
result = func(*args, **kwargs)
logging.info(f"{func.__name__} finished execution")
return result
return wrapper
C. Create reusable wrappers for common tasks
Decorators excel at creating reusable wrappers for common tasks. Here's a comparison of some useful reusable decorators:
Decorator | Purpose | Example Use Case |
---|---|---|
@retry | Automatically retry a function on failure | API calls with potential network issues |
@memoize | Cache function results for repeated calls | Expensive computations with same inputs |
@rate_limit | Limit the number of function calls in a given time period | Prevent API abuse or overuse |
@deprecated | Mark functions as deprecated and warn users | Maintain backwards compatibility while encouraging updates |
By mastering decorators, you can significantly enhance your code's functionality and maintainability. Next, we'll explore advanced string formatting techniques to further refine your Python skills.
Exploring Advanced String Formatting

Master f-strings for cleaner code
F-strings, introduced in Python 3.6, offer a concise and readable way to embed expressions inside string literals. They provide a significant improvement over older formatting methods, making your code cleaner and more maintainable.
Key advantages of f-strings:
-
Simplicity: Directly embed variables and expressions
-
Performance: Faster than other string formatting methods
-
Readability: Easy to understand at a glance
Example usage:
name = "Alice"
age = 30
print(f"Hello, {name}! You are {age} years old.")
Use format() method for complex string operations
While f-strings are great for simple cases, theformat()
method excels in more complex scenarios, offering fine-grained control over string formatting.
Benefits of format():
-
Reusability: Use the same format string multiple times
-
Flexibility: Supports positional and keyword arguments
-
Precision control: Easily format numbers and dates
Feature | Example |
---|---|
Positional | "{0} is {1} years old".format(name, age) |
Keyword | "{name} is {age} years old".format(name=name, age=age) |
Alignment | "{:>10}".format("right") # Right-aligned in 10 spaces |
Implement custom string formatting
For advanced use cases, Python allows you to define custom string formatting behavior for your objects. This is particularly useful when working with complex data structures or domain-specific types.
Steps to implement custom formatting:
-
Define a
__format__()
method in your class -
Specify how the object should be formatted
-
Use the format specifier in f-strings or format() method
Now that we've explored advanced string formatting techniques, let's move on to optimizing our code with context managers.
Optimizing with Context Managers

Ensure proper resource management
Context managers in Python are powerful tools for efficient resource management. They automatically handle the setup and teardown of resources, ensuring proper allocation and release. This is particularly useful for file handling, database connections, and network sockets.
Here's a comparison of resource management with and without context managers:
Without Context Manager | With Context Manager |
---|---|
Manually open and close | Automatic cleanup |
Risk of resource leaks | Guaranteed release |
More error-prone code | Cleaner, safer code |
Requires try-finally blocks | Simplified syntax |
Create custom context managers
Creating custom context managers is straightforward using thecontextlib
module or by implementing the__enter__
and__exit__
methods. This allows you to define specific behavior for resource acquisition and release.
Example of a custom context manager:
from contextlib import contextmanager
@contextmanager
def custom_context():
print("Entering context")
yield
print("Exiting context")
Simplify file handling and database operations
Context managers excel in simplifying file handling and database operations. They ensure that files are properly closed and database connections are released, even if exceptions occur.
-
File handling example:
with open('file.txt', 'r') as file:
content = file.read()
# File is automatically closed after the block
-
Database operation example:
with database.connection() as conn:
cursor = conn.cursor()
cursor.execute("SELECT * FROM table")
results = cursor.fetchall()
# Connection is automatically closed after the block
By leveraging context managers, you can write cleaner, more efficient code while minimizing the risk of resource leaks. This optimization technique is crucial for writing robust and maintainable Python applications.
Unlocking the Potential of *args and **kwargs

Create flexible function signatures
Python's*args
and**kwargs
allow you to create incredibly flexible function signatures. These powerful features enable you to write functions that can accept any number of positional and keyword arguments.
-
*args
: Captures an arbitrary number of positional arguments -
**kwargs
: Captures an arbitrary number of keyword arguments
Here's a simple example demonstrating their use:
def flexible_function(*args, **kwargs):
print(f"Positional arguments: {args}")
print(f"Keyword arguments: {kwargs}")
flexible_function(1, 2, 3, name="Alice", age=30)
Unpack arguments dynamically
One of the most powerful aspects of*args
and**kwargs
is their ability to unpack arguments dynamically. This feature is particularly useful when you need to pass arguments to another function or when working with functions that have varying parameter requirements.
Operation | Syntax | Description |
---|---|---|
Unpacking lists | *args |
Expands a list into individual arguments |
Unpacking dictionaries | **kwargs |
Expands a dictionary into keyword arguments |
Example of dynamic unpacking:
def greet(name, age):
print(f"Hello, {name}! You are {age} years old.")
person_info = ["Alice", 30]
greet(*person_info)
person_dict = {"name": "Bob", "age": 25}
greet(**person_dict)
Implement function wrappers and decorators
*args
and**kwargs
are instrumental in creating flexible function wrappers and decorators. They allow you to create wrappers that can work with any function, regardless of its signature.
Here's a simple timing decorator using*args
and**kwargs
:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute.")
return result
return wrapper
@timing_decorator
def slow_function(n):
time.sleep(n)
slow_function(2)
This powerful combination of*args
and**kwargs
allows you to create more adaptable and reusable code, making your Python programs more flexible and maintainable.
Mastering Dictionary Tricks
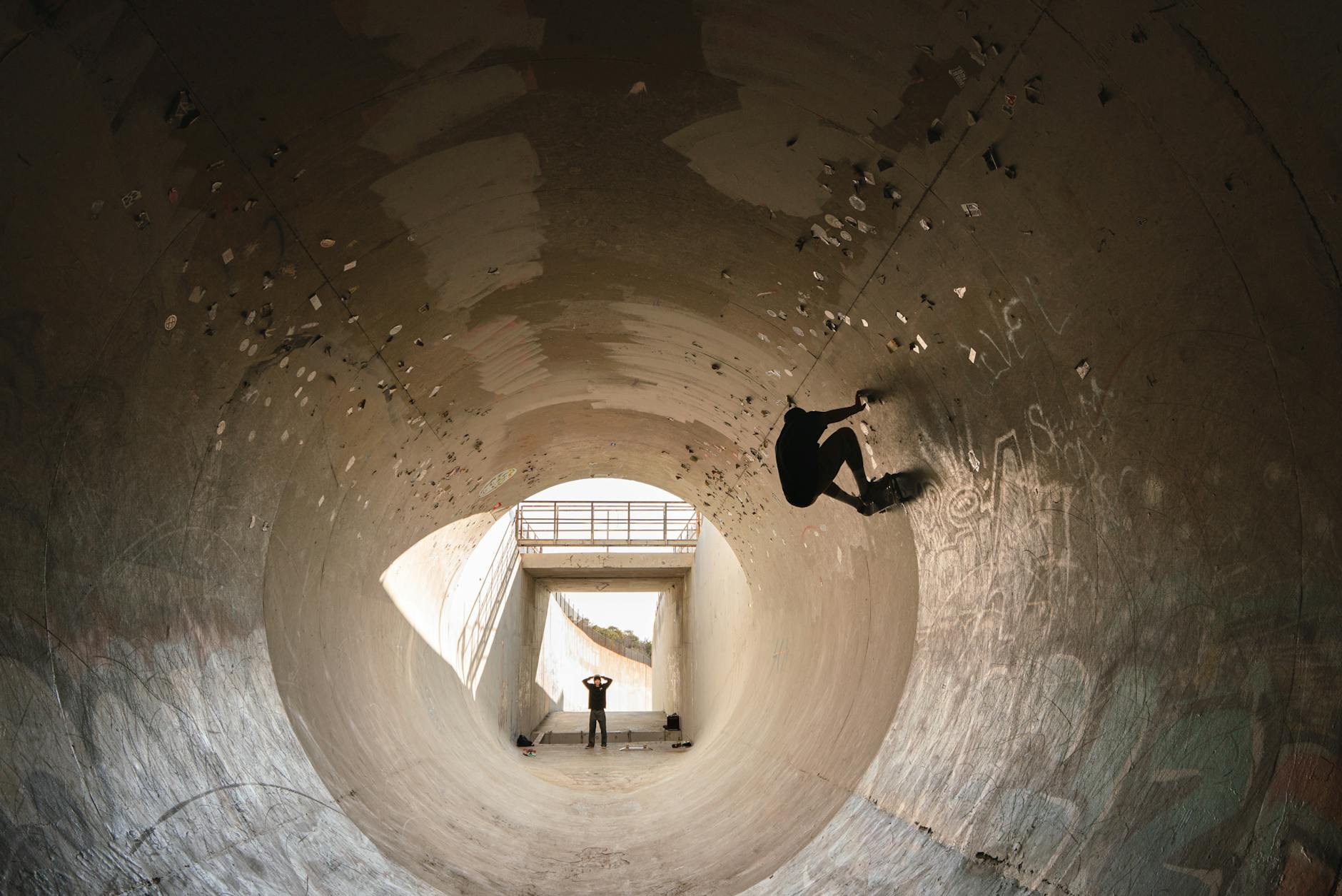
Merge dictionaries efficiently
Python offers several efficient ways to merge dictionaries. One of the most elegant methods is using the|
operator introduced in Python 3.9. This approach combines two or more dictionaries into a new one without modifying the originals.
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = dict1 | dict2
For earlier Python versions, you can use theupdate()
method or the**
unpacking operator:
# Using update()
merged_dict = dict1.copy()
merged_dict.update(dict2)
# Using ** operator
merged_dict = {**dict1, **dict2}
Use defaultdict for automatic key initialization
Thedefaultdict
class from thecollections
module is a powerful tool for handling missing keys. It automatically initializes new keys with a default value, eliminating the need for explicit key checks.
from collections import defaultdict
# Example: Counting word frequencies
word_count = defaultdict(int)
text = "the quick brown fox jumps over the lazy dog"
for word in text.split():
word_count[word] += 1
print(word_count)
Method | Use Case |
---|---|
Regular dict | General purpose |
defaultdict | Automatic initialization |
Counter | Counting occurrences |
Implement ordered dictionaries
Since Python 3.7, regular dictionaries maintain insertion order by default. However, for explicit ordered dictionaries, useOrderedDict
from thecollections
module:
from collections import OrderedDict
ordered_dict = OrderedDict()
ordered_dict['first'] = 1
ordered_dict['second'] = 2
ordered_dict['third'] = 3
print(list(ordered_dict.items()))
These dictionary tricks can significantly enhance your Python coding skills, making your code more efficient and readable.
Leveraging Python's Built-in Functions
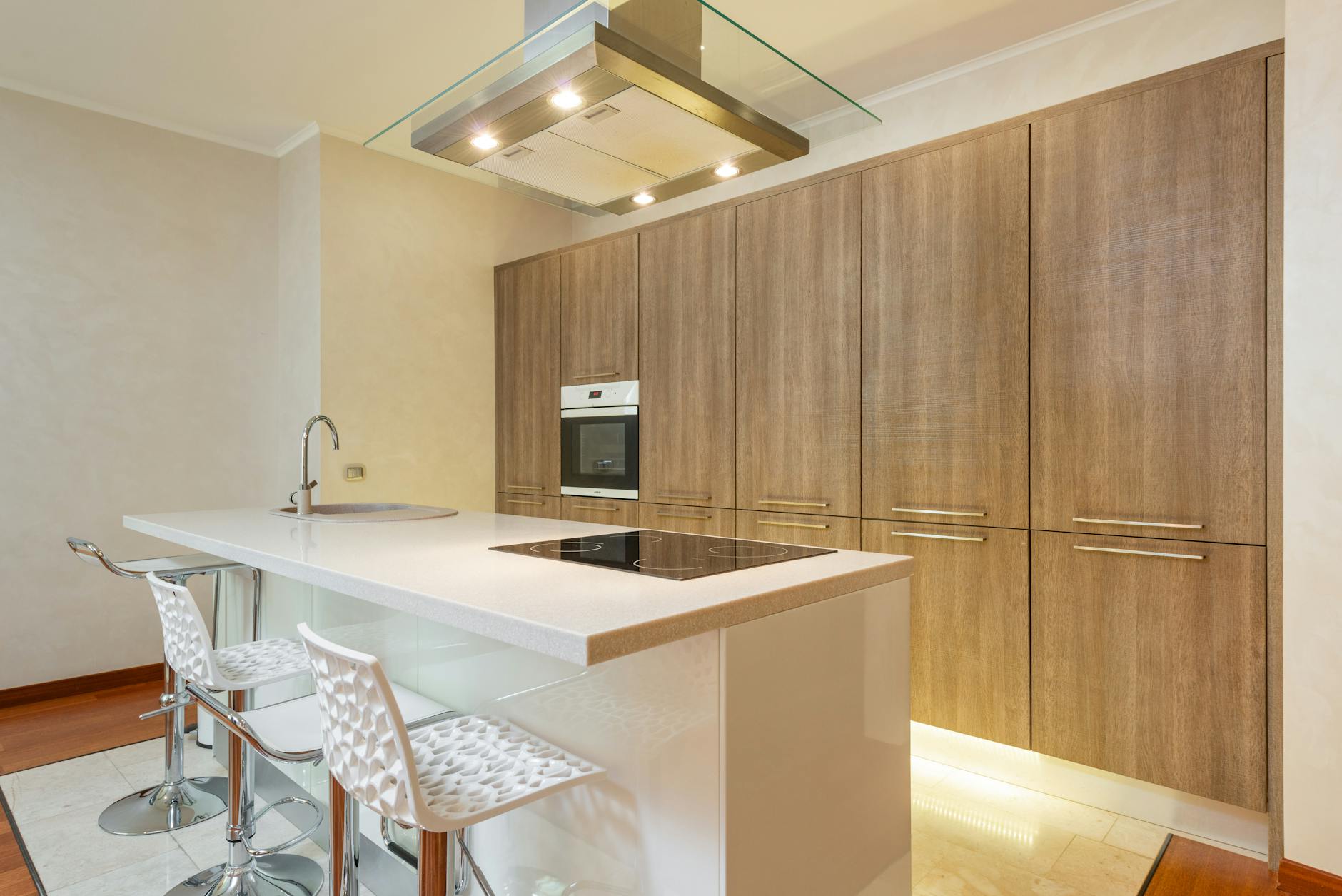
Simplify code with map() and filter()
Python's built-in functionsmap()
andfilter()
are powerful tools for streamlining your code.map()
applies a function to each item in an iterable, whilefilter()
selects items from an iterable based on a condition.
# Example using map()
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16, 25]
# Example using filter()
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
Use zip() for parallel iteration
Thezip()
function allows you to iterate over multiple iterables simultaneously, creating tuples of corresponding elements.
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
for name, age in zip(names, ages):
print(f"{name} is {age} years old")
Employ enumerate() for indexed loops
enumerate()
adds a counter to an iterable, making it easy to access both the index and value in a loop.
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"Index {index}: {fruit}")
Harness the power of any() and all()
any()
andall()
are useful for checking conditions across iterables.any()
returns True if any element is True, whileall()
returns True if all elements are True.
Function | Description | Example |
---|---|---|
any() |
Returns True if any element is True | any([False, True, False]) returns True |
all() |
Returns True if all elements are True | all([True, True, True]) returns True |
Now that we've explored these built-in functions, let's move on to embracing Pythonic idioms for even more efficient coding.
Embracing Pythonic Idioms

Write more readable and efficient code
Embracing Pythonic idioms is essential for writing clean, efficient, and readable code. Let's explore some key idioms that will elevate your Python programming skills:
Use 'if x in list' instead of loops
One of the most elegant Pythonic idioms is the use of the 'in' operator for membership testing. This approach is not only more readable but also more efficient than using loops.
Traditional Approach | Pythonic Approach |
---|---|
for item in list: if item == x: return True |
if x in list: return True |
Implement slicing for advanced data manipulation
Slicing is a powerful Pythonic idiom that allows for efficient manipulation of sequences like lists and strings.
-
Basic slicing:
my_list[start:end]
-
Step slicing:
my_list[start:end:step]
-
Reverse slicing:
my_list[::-1]
Utilize the 'else' clause in loops
Python uniquely offers an 'else' clause for loops, which executes when the loop completes without encountering a 'break' statement.
for item in items:
if condition:
break
else:
print("Loop completed without breaking")
This idiom is particularly useful for search operations and can replace flag variables often used in other languages.
Now that we've covered these Pythonic idioms, let's move on to explore how you can leverage Python's built-in functions to further enhance your coding efficiency.
Python's versatility and power shine through these ten advanced techniques. By mastering list comprehensions, lambda functions, generators, decorators, and advanced string formatting, you'll write more efficient and elegant code. Utilizing context managers, *args and **kwargs, dictionary tricks, built-in functions, and Pythonic idioms will further elevate your programming skills.
Embrace these Python tricks and watch your coding abilities soar. As you incorporate them into your projects, you'll find yourself writing cleaner, more efficient, and more sophisticated code. Remember, becoming a coding wizard is a journey of continuous learning and practice. Keep exploring, experimenting, and pushing the boundaries of what you can achieve with Python!